Introduction
React is a popular JavaScript library for building user interfaces, and it's widely used in web development today. If you're new to React, don't worry; this blog post will provide you with a basic introduction and some hands-on examples to get you started.
Why is React
React is an open-source library created by Facebook that allows developers to build dynamic and interactive user interfaces for web applications. It's known for its component-based architecture, which makes it easy to create reusable UI elements.
Setting Up React
To begin working with React, you'll need Node.js and npm (Node Package Manager) installed on your system. You can create a new React project using a tool called "Create React App." Here's a simple example:
npx create-react-app my-react-app
cd my-react-app
npm start
Creating Your First React Component
In React, everything is a component. Let's create a simple "HelloWorld" component:
import React from 'react';
function HelloWorld() {
return <h1>Hello, World!</h1>;
}
export default HelloWorld;
You can use this component in your application by importing and rendering it within another component or the main application file.
Rendering React Components
Here's how you can render the "HelloWorld" component in your App.js file:
import React from 'react';
import HelloWorld from './HelloWorld';
function App() {
return (
<div className="App">
<HelloWorld />
</div>
);
}
export default App;
In this example, we import the "HelloWorld" component and include it within the App component's render method. When you run your React application, you'll see "Hello, World!" displayed in your browser.
JSX: The Power of HTML in JavaScript
React uses JSX (JavaScript XML) to define its components. JSX allows you to write HTML-like code directly within your JavaScript files. This makes it easy to visualize and create user interface elements.
const element = <h1>Hello, JSX!</h1>;
JSX is not actual HTML but a syntax extension. It is transpiled by tools like Babel into regular JavaScript that the browser can understand.
Props: Passing Data to Components
Props (short for "properties") are a fundamental concept in React. They allow you to pass data from a parent component to a child component. Let's modify our "HelloWorld" component to accept a name prop and personalize the greeting:
import React from 'react';
function HelloWorld(props) {
return <h1>Hello, {props.name}!</h1>;
}
export default HelloWorld;
Now, you can pass a name prop when using the "HelloWorld" component:
<HelloWorld name="Alice" />
<HelloWorld name="Bob" />
This will render "Hello, Alice!" and "Hello, Bob!" in your application.
State: Managing Component Data
In addition to props, React provides a concept called "state" for managing a component's internal data. State allows components to store and update information that can change over time. Whatever there is in state, reflected on UI.
Let's create a simple counter component using state:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
In this example, we use the useState hook to create a count state variable, initialized to 0. We also define an increment function that updates the count when the button is clicked. So, when we want to update number and display the latest one, we just update the state and it will automatically be rendered on UI.
Lifecycle Methods and Effects
React components have lifecycle methods that allow you to perform actions at various stages of a component's life, such as when it is created, updated, or destroyed. In modern React, you can use the useEffect hook to manage side effects inside React Functional Components (RFC).
Here's an example of using useEffect to fetch data when a component mounts:
import React, { useState, useEffect } from 'react';
function DataFetcher() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then((response) => response.json())
.then((result) => setData(result))
.catch((error) => console.error(error));
}, []);
return (
<div>
{data ? (
<p>Data: {data}</p>
) : (
<p>Loading...</p>
)}
</div>
);
}
export default DataFetcher;
In this example, the useEffect hook is used to fetch data from an API when the component mounts. The [] passed as the second argument ensures that the effect runs only once, similar to the componentDidMount lifecycle method in class-based components. We can add dependencies inside [] if we want to trigger re-render.
Handling Events
React makes it easy to handle user interactions by using event handlers. Here's an example of handling a button click event:
import React, { useState } from 'react';
function ClickCounter() {
const [count, setCount] = useState(0);
const handleClick = () => {
setCount(count + 1);
};
return (
<div>
<p>Click Count: {count}</p>
<button onClick={handleClick}>Click Me</button>
</div>
);
}
export default ClickCounter;
In this component, we define a handleClick function that updates the count state when the button is clicked. We then attach this function to the button's onClick event.
Conditional Rendering
React allows you to conditionally render components or elements based on certain conditions. This is useful for showing or hiding parts of your UI dynamically. Here's an example of conditional rendering:
import React, { useState } from 'react';
function ToggleContent() {
const [showContent, setShowContent] = useState(false);
return (
<div>
<button onClick={() => setShowContent(!showContent)}>
Toggle Content
</button>
{showContent && <p>This content is now visible!</p>}
</div>
);
}
export default ToggleContent;
In this example, we use the showContent state to conditionally render a <p> element. When the "Toggle Content" button is clicked, it toggles the showContent state, resulting in the content being shown or hidden.
Lists and Keys
React makes it easy to render lists of items by using the map function along with unique keys to identify each item in the list. Here's an example:
import React from 'react';
function ListExample() {
const items = ['Item 1', 'Item 2', 'Item 3'];
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
}
export default ListExample;
In this example, we use the map function to iterate over the items array and generate a list of <li> elements. We use the key prop to provide a unique identifier for each item.
Forms and Controlled Components
React allows you to create controlled form components, where the form input values are controlled by the component's state. Here's an example of a simple controlled form:
import React, { useState } from 'react';
function ControlledForm() {
const [formData, setFormData] = useState({
username: '',
email: '',
});
const handleChange = (e) => {
const { name, value } = e.target;
setFormData({
...formData,
[name]: value,
});
};
const handleSubmit = (e) => {
e.preventDefault();
// Submit the form data
console.log(formData);
};
return (
<form onSubmit={handleSubmit}>
<div>
<label htmlFor="username">Username:</label>
<input
type="text"
id="username"
name="username"
value={formData.username}
onChange={handleChange}
/>
</div>
<div>
<label htmlFor="email">Email:</label>
<input
type="email"
id="email"
name="email"
value={formData.email}
onChange={handleChange}
/>
</div>
<button type="submit">Submit</button>
</form>
);
}
export default ControlledForm;
In this example, we use the formData state to control the input fields. The handleChange function updates the state whenever the input values change, and the handleSubmit function is called when the form is submitted.
Styling in React
React doesn't prescribe a specific way to style components, but you can use various methods to apply styles:
Inline Styles: You can apply styles directly to JSX elements using the `style` prop.
<div style={{ backgroundColor: 'blue', color: 'white' }}>Styled Div</div>
CSS Modules: You can use CSS Modules, which allow you to write CSS files where class names are scoped to the component.
import styles from './MyComponent.module.css';
function MyComponent() {
return <div className={styles.myComponent}>Styled Div</div>;
}
Styled-Components: You can use a library like styled-components to write CSS-in-JS.
import styled from 'styled-components';
const StyledDiv = styled.div`
background-color: blue;
color: white;
`;
function MyComponent() {
return <StyledDiv>Styled Div</StyledDiv>;
}
Choose the styling approach that best suits your project's requirements.
React Router: Navigating Between Pages
For building single-page applications (SPAs) with multiple views or pages, you can use the React Router library. Here's a basic example of setting up React Router:
import React from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function Home() {
return <h1>Home Page</h1>;
}
function About() {
return <h1>About Page</h1>;
}
function App() {
return (
<Router>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
</ul>
</nav>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</Router>
);
}
export default App;
In this example, we import components from React Router and define routes for the Home and About pages. The <Link> component is used to navigate between pages.
Context API: Managing Global State
When your application grows, you may need a way to manage global state. React's Context API provides a way to share state among components without passing props manually through every level of your component tree.
Here's a simplified example of using the Context API to manage a theme:
import React, { createContext, useContext, useState } from 'react';
const ThemeContext = createContext();
function ThemeProvider({ children }) {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme(theme === 'light' ? 'dark' : 'light');
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
}
function ThemeToggleButton() {
const { theme, toggleTheme } = useContext(ThemeContext);
return (
<button onClick={toggleTheme}>
Toggle Theme ({theme})
</button>
);
}
function App() {
return (
<ThemeProvider>
<div>
<h1>Theme Example</h1>
<ThemeToggleButton />
</div>
</ThemeProvider>
);
}
export default App;
In this example, the `ThemeContext` is created and used by the `ThemeProvider` and `ThemeToggleButton` components to manage and toggle the theme state.
Redux: State Management for Larger Applications
For more complex applications, you may want to consider using Redux for state management. Redux provides a predictable way to manage and update the application's state.
Here's a high-level overview of Redux:
- Store: The store holds the application's state.
- Actions: Actions describe what happened in the application.
- Reducers: Reducers specify how the application's state changes in response to actions.
- Dispatch: Dispatching an action is the only way to change the state.
Implementing Redux in your React application involves setting up a store, defining actions, reducers, and connecting components to the store using libraries like react-redux.
Testing in React
Testing is a crucial part of the development process. React applications can be tested using various testing libraries and frameworks, such as Jest, React Testing Library, and Enzyme. These tools allow you to write unit tests and integration tests to ensure your components and application work as expected.
Here's a simple example of a unit test for a React component using Jest and React Testing Library:
import React from 'react';
import { render, screen } from '@testing-library/react';
import HelloWorld from './HelloWorld';
test('renders a greeting', () => {
render(<HelloWorld />);
const greetingElement = screen.getByText(/Hello, World!/i);
expect(greetingElement).toBeInTheDocument();
});
In this example, we render the "HelloWorld" component and use screen.getByText to find the greeting text and assert its presence.
Conclusion
This comprehensive guide has taken you from the basics of React to more advanced topics like state management, routing, and testing. React's component-based architecture, along with its ecosystem of libraries and tools, empowers developers to build dynamic and interactive web applications efficiently.
As you continue your journey with React, explore documentation, tutorials, and real-world projects to deepen your understanding and become a proficient React developer. Remember that practice and hands-on experience are key to mastering React and building amazing web applications. Happy coding!
Binod Chaudhary
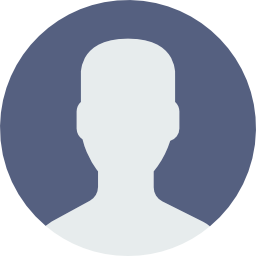
Software Engineer | Full-Stack Developer