Background
When we are developing real-world projects, file management is one of the crucial parts of a project. For projects like online shopping, renting services, where image files grow rapidly over time, it becomes too complex and overhead if it is stored on the same server with the project. Because files are usually large in size and can occupy most of the server space and request/response overhead.
Also, it is good practise to manage files and project codes on different servers. There are plenty of services that provide cloud storage for files and assets. AWS S3 and Cloudinary are one of the leading services out there.
In this tutorial, we are going to use Cloudinary service, since it's free and does not require a credit card for basic use.
So before we begin, make sure you have created an account in cloudinary and get your API keys. Follow the link below to create an account.
Get API Keys and Secret
After creating an account get the following details from cloudinary dashboard.
- API key
- API secret
- Cloud name
I am assuming, you are implementing cloudinary API in node js project. So, I am not setting up complete node js app here to make this tutorial short and simple.
So, we are going to create a function that will take an image file path as input and upload that image to cloudinary server. Before we write our function, install npm packages
npm install cloudinary --save
npm install body-parser --save
// body-parser is used to parse form data in express js
Now, let's create our upload function.
const cloudinary = require("cloudinary").v2;
const fs = require("fs");
cloudinary.config({
cloud_name: YOUR_CLOUD_NAME,
api_key: YOUR_API_KEY,
api_secret: YOUR_API_SECRET
});
const uploadToCloud = filePath => {
return new Promise((resolve, reject) => {
cloudinary.uploader
.upload(filePath)
.then(result => {
fs.unlinkSync(filePath);
// Remove uploaded file from our server once it gets uploaded to cloudinary server.
resolve(result);
})
.catch(error => {
reject(error);
});
});
};
Explanation:
- First of all, we imported cloudinary and set config variables.
- Then created a javascript promise function that takes an input argument i.e. the absolute path of the image to be uploaded.
- If the image is uploaded,
resolve
block will be executed, otherwisereject
block will be executed. - We can call this function where-ever we want, we just need to pass the absolute path of an image to be uploaded.
To get an absolute path of an image uploaded, we can use multer npm package
as follows:
const multer = require("multer");
const path = require("path");
const upload = multer({
storage: multer.diskStorage({
destination: "./public/uploads/", // path to directory inside a project
filename: function(req, file, cb) {
cb(
null,
"myfile-" + Date.now() + path.parse(file.originalname).ext
);
}
})
});
router.post("/upload-image", upload.single("imageFile"), (req, res, next) => {
uploadToCloud(req.file.path)
.then(d => res.json(d))
.catch(e => next(e));
});
So that's all. you can now call /upload-image
API with the field name imageFile
using postman or your frontend service.
Note: Make sure your node app is well set up to handle routes requests and form data with your node framework. eg: Express JS
Binod Chaudhary
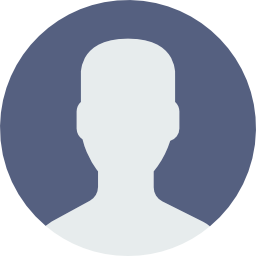
Software Engineer | Full-Stack Developer