Introduction
JSON web token(JWT) is basically a digitally signed string, in which some data is encoded and can be transmitted over the network.
Let's make it simpler.
You can think of it as a piece of paper with an authorized signature. Let us assume the paper is signed by education ministry (government) so that you can get admission to any government school or college.
If you haven't worked on it before, JWT token looks like this:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiYWRtaW4iOnRydWV9.dyt0CoTl4WoVjAHI9Q_CwSKhl6d_9rhM3NrXuJttkao
If you look at it closely, you can find two dots(.) dividing it into three sections. These three individual sections have different values.
Components
1. Header:
The first part of the token is a header which is base64Url. It holds the meta-information of a token which can be used by a receiver in order to validate the token.
If we decode only the first portion of a header, it will provide the JSON object as follows.
{
"alg": "HS256",
"typ": "JWT"
}
We can see, this is just a plain object, and the signature algorithm is "HS256" for this particular token.
2. Payload:
The payload, also knows as the claim is information about an entity like user info. Furthermore, it also includes metadata of that particular payload as follows:
iat
- Timestamp of the issued date.sub
- Technical identifier of the user.exp
- JWT expiration time.iss
- The issuer of the token.
Upon decoding a payload only i.e. middle part of a token, we get the entity information (eg: user entity) as follows:
{
"sub": "1234567890",
"firstname": "John",
"lastname": "Doe",
"admin": true,
"iat": 1516239022
}
And these are the claims we are going to send to servers that maybe our own server or any third-party server that supports JWT.
3. Signature:
The final token is generated by applying JWS (JSON Web Specification). The final part of JWT is generated by combining encoded JWT header, encoded JWT payload, and signing algorithms like HMAC SHA-256.
Since we know the basics of JWT now, let's create a token. I am using node js for this tutorial.
const jwt = require("jsonwebtoken");
const secrete_key = 'your_secret_key'
const user = { _id : 1234, firstname: "John", lastname: "Doe" }
const _token = jwt.sign(user.toJSON(), secret_key, {
expiresIn: 60 * 60 * 24, // 24 hours
});
What we did above:
- Imported
jsonwebtoken
as jwt from the npm package. - Defined a secret key to sign token. Remember that, the same key is used to decode token so don't make it public.
- Set user details, it can be logged in user information in case of real applications.
- Signed a token with
jwt.sign()
method with payload, secret key, and expiration time.
So far we have created a token, but we need to verify it before we provide any access to server resources using this token. Look at the code below to verify JWT.
const jwt = require("jsonwebtoken");
const SECRET_KEY = "same_secret_key_used_to_sign_token";
jwt.verify(token, SECRET_KEY, function (err, token_data) {
if (err) throw err;
console.log("Verified token data:", token_data);
});
Simple as that. Just call a jwt.verify()
function with a secret key and you should have your payload data i.e. user data in this case.
Summary:
- JWT is an authorization token to make sure someone has access to some resource or entity.
- It has three basic components; Header, Payload, and Signature.
- It can be decrypted if anybody intercepts the token. So don't put private information like credit card details, bank account details, and all in it.
- JWT becomes very useful in micro-service architecture where we can do request/response between multiple servers. In traditional systems, logged in user details were stored in session which is valid only in, that one particular server it was created.
- It is encoded as base64Url so that it becomes reliable to transfer over different servers. Other string formats may invite issues like, automatically concatenating special characters or symbols.
- If expiring time is not specified while signing the token, JWTs never expire.
That brings us to an end. I hope it was helpful enough to understand about JWT. Keep learning, keep sharing!
Binod Chaudhary
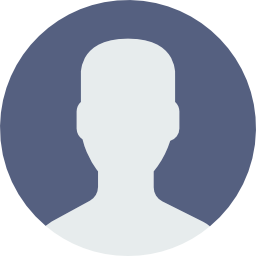
Software Engineer | Full-Stack Developer